Nesting Lists
Nesting multiple Probability Lists within other collections significantly enhances organizational flexibility and functionality.
Using our specialized class, PLCollection
, over standard C# List or Array, brings additional benefits.
These include the ability to name lists within the collection and select values from the entire collection, enhancing both usability and functionality.
This page introduces the features and usage of PLCollection class. For practical implementation guides, visit the PLCollection Guides page.
PLCollection Class
The PLCollection<T>
, short for Probability List Collection, is a generic class designed to hold multiple Probability Lists of the same type T
.
This class offers useful methods that help manage these lists and also enables the selection of values from the entire collection.
For game designers, PLCollection
provides a significant advantage with its special inspector drawer in the Unity Editor. This feature makes it easier to manage and visualize the lists, enhancing the design process.
Setting up PLCollection
To begin organizing your lists, simply declare a PLCollection<T>
within your MonoBehaviour or ScriptableObject scripts. This is done in a manner similar to declaring a ProbabilityList
of your chosen type.
Adding PLCollection
using UnityEngine;
using RNGNeeds;
public class MyScript : MonoBehaviour
{
public PLCollection<string> myCollection;
}
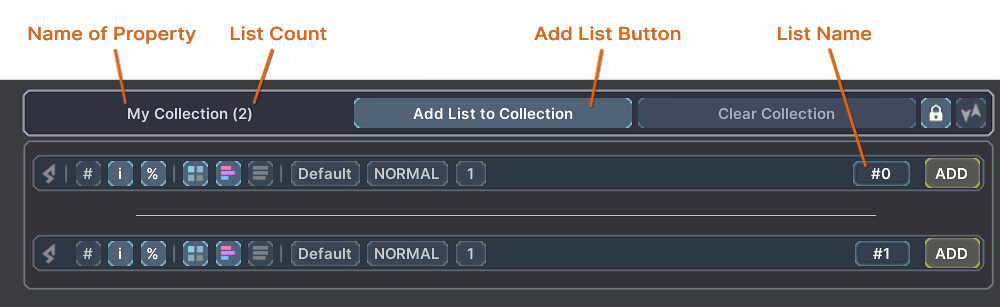
In the Unity Editor Inspector, you will notice a new section for PLCollection
.
At the top left, the name of your property is displayed, alongside the number of lists currently in the collection.
Lists can be added by clicking the Add List to Collection button.
Naming Lists
Newly created lists within the PLCollection
are automatically assigned a name that corresponds to their position in the collection.
The default format for these names is #index
, such as #0
, #1
, #2
, and so forth.
You can easily rename any list by clicking on the name field and entering a new name.
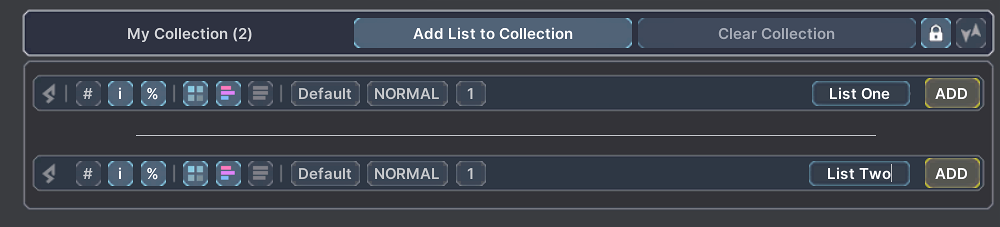
The name is stored within the ProbabilityList
object itself, rather than in the PLCollection
class.
This allows flexibility in naming as you can set the ListName
property for any list, regardless of whether it is part of a collection.
myProbabilityList.ListName = "My List";
If a list is added without a specified name, PLCollection
will automatically assign a default name based on its position.
Adding and naming lists via code
// Adding an unnamed list
myCollection.AddList(myList); // List Name will be '#0'
myCollection.AddList(myList, "My List"); // List Name will be 'My List'
// Adding a named list
myList.ListName = "My List";
myCollection.AddList(myList); // List Name will not change
// Renaming a list
myCollection.SetListName(0, "My List");
myCollection.SetListName("My List", "New Name");
The SetListName
method returns true
if the list was found and renamed successfully, and false
otherwise.
Reordering Lists
To enable reordering of lists within the inspector, click the Reorder Lists button located in the top right corner of the collection. Arrows will then appear next to each list, allowing you to adjust their order by moving them up or down.
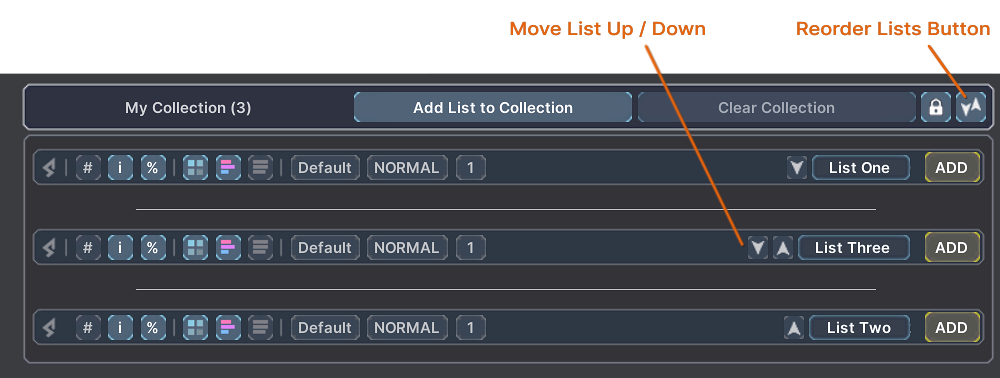
Lists can also be reordered programmatically using the MoveListUp
and MoveListDown
methods.
Reordering lists via code
myCollection.MoveListUp(1); // Move list at index 1 up
myCollection.MoveListDown(1); // Move list at index 1 down
A list can only be moved if it is not already the first or last in the collection.
The methods MoveListUp
and MoveListDown
will return true
if the list was successfully moved, and false
otherwise.
Removing Lists
The collection is Locked by default to prevent accidental removal of lists. To enable list removal, click the Lock button located in the top right corner of the collection.
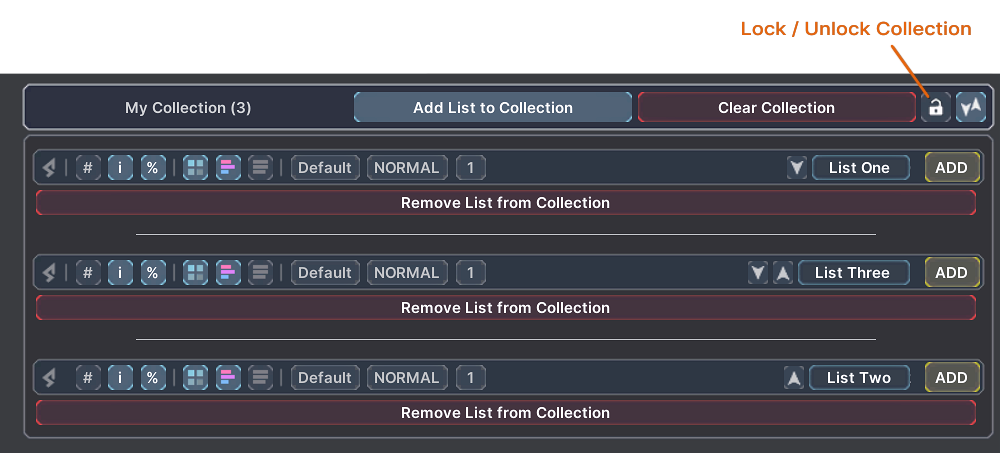
Once unlocked, red Remove List from Collection buttons will appear next to each list, allowing you to easily delete them. Additionally, the entire collection can be cleared by clicking the Clear Collection button found in the header.
Removing a list or clearing the collection are significant actions, underscored by the red color of the buttons. Although these actions do not trigger a confirmation popup, they can be reversed using the Unity Editor's Undo feature.
Removing lists via code
myCollection.RemoveList(1); // Remove list at index 1
myCollection.RemoveList("My List"); // Remove list by name "My List"
myCollection.ClearCollection(); // Removes all lists from the collection
The RemoveList
methods return true
if the list was successfully found and removed, and false
otherwise.
Clearing Lists
PLCollection also provides handy methods for clearing the individual lists, i.e., removing all items from a list. This feature is useful when you want to maintain the structural framework of the collection but eliminate the contents of the lists. Note that clearing a list will also remove its History. For more details on History, refer to the Pick History section.
Clearing lists via code
myCollection.ClearList(1); // Clear list at index 1
myCollection.ClearList("My List"); // Clear list by name "My List"
myCollection.ClearAllLists(); // Clear all lists in the collection
The ClearList
methods return true
if the list was successfully found and cleared, and false
otherwise.
Selecting Values from Collection
The PLCollection class provides a set of methods for selecting values from individual lists or the entire collection. While the collection itself does not handle the selection logic, it offers a convenient layer for managing and accessing multiple lists.
To select values from a specific list in the collection, you can retrieve the list directly or utilize the methods provided by PLCollection.
Selecting Directly from Probability List
Access a list from the collection either by its index or name, and then select a value from it as you would with any standard Probability List. For more information on how to select values from a list, visit the Selecting Values page.
Selecting values directly from list
// Retrieving list from collection
var list = myCollection.GetList(0); // Get list at index 0
if (myCollection.GetList("MyList", out var list)) // Get list by name "MyList"
{
var value = list.PickValue();
}
Selecting via PLCollection
The PLCollection class provides a set of methods for selecting values from the lists within. These methods are named after the corresponding methods in the ProbabilityList class and their summary clearly indicates which method is used and how are the results returned.
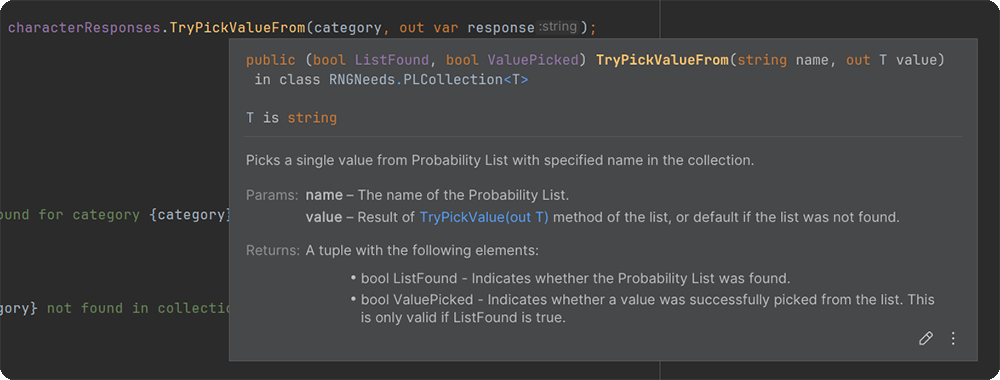
It's important to understand the returns when using these methods, as some operations in ProbabilityList
check if a value was successfully picked.
On top of this, PLCollection
adds a layer that checks whether the list itself was found.
Since picking a value from a list is not guaranteed—the list may be empty or contain disabled items—it's crucial to know both if the list was found and if a value was successfully picked.
Determining the success of a pick is especially important with value types, where the default value might be returned in case of an unsuccessful pick.
Excerpt from Selecting Values page:
"However, if you are working with value types
, the result of an unsuccessful pick would be the default
value of your Type.
In some cases, the value returned from an unsuccessful pick might be indistinguishable from a real one.
For example, with value types such as string
, boolean
, or int
, an empty string, a false
, or a 0
would be returned, respectively."
Here are the various families of methods available for picking values from the collection:
- Pick Value From - Methods that pick a single value from a list:
bool PickValueFrom(int index, out T value)
bool PickValueFrom(string name, out T value)
- Try Pick Value From - Methods that pick a single value from a list and return a tuple indicating whether the list was found and whether the value was picked:
(bool ListFound, bool ValuePicked) TryPickValueFrom(int index, out T value)
(bool ListFound, bool ValuePicked) TryPickValueFrom(string name, out T value)
- Pick Values From (simplified variants) - Methods that pick multiple values from a list. These methods return a list of values, and they do not indicate whether a value was picked. If the list was not found, the result is null, and if no values were selected, the list is empty:
List<T> PickValuesFrom(int index)
List<T> PickValuesFrom(string name)
- Pick Values From (full variants) - Methods that pick multiple values from a list and return a boolean indicating whether the list was found:
bool PickValuesFrom(int index, out List<T> values)
bool PickValuesFrom(string name, out List<T> values)
- Pick Values From (fill variants) - Methods that pick multiple values from a list and fill an existing list with the results. These methods also return a boolean indicating whether the list was found:
bool PickValuesFrom(int index, List<T> listToFill)
bool PickValuesFrom(string name, List<T> listToFill)
- Pick Value From All - Method that picks a single value from each list in the collection:
List<T> PickValueFromAll()
- Pick Values From All - Method that picks multiple values from each list in the collection:
List<T> PickValuesFromAll()
With the addition of Depletable Lists in RNGNeeds v0.9.7, the PLCollection
class also includes methods for refilling units of all lists in the collection:
- Refill List - Methods that refill the units of a list in the collection:
bool RefillList(int index)
bool RefillList(string name)
- Refill All Lists - Method that refills the units of all lists in the collection:
void RefillAllLists()
Example: Selecting Values via PLCollection
Here is an example demonstrating how to select values from a list within the collection using the TryPickValueFrom
method.
Selecting values via PLCollection
var result = myCollection.TryPickValueFrom(0, out var value);
result.ListFound // Indicates whether the list was found
result.ValuePicked // Indicates whether the value was picked
if (myCollection.TryPickValueFrom("My List", out var value).ValuePicked)
{
// Value was picked, therefore list was found and not empty
}
else
{
// Value was not picked - the selection was unsuccessful
// could also mean the list was not found or was empty
}
Summary
The PLCollection class streamlines the management of Probability Lists in Unity, enabling easy organization, modification, and value selection from multiple lists. It offers practical features like list naming, reordering, and removal, coupled with a variety of methods for accessing values efficiently.
For a practical use cases, explore the
PLCollection Guides, which provides a detailed guide for implementing PLCollection
in your projects.
For more complex use cases utilizing a modular loot table approach, check out the Treasure Chest and Deck Builder samples included with the plugin.
Learn more about these and other examples on the Samples Overview.