PLCollection Guides
These guides are designed to help you implement and utilize the PLCollection class for nesting and organizing Probability Lists in your Unity projects. To learn more about PLCollection, visit the Nesting Lists page of our documentation.
Have a use case in mind that we should explore on this page? Join our Discord community and share your thoughts.
Collection of NPC Responses
Let's create a simple example where we have a collection of responses for NPC characters, organizing them into different categories.
First, create a MonoBehaviour class called CharacterResponses
that will hold our collection of responses.
We will include method SelectResponse
that will select a response from a specific category.
CharacterResponses.cs
using UnityEngine;
using RNGNeeds;
public class CharacterResponses : MonoBehaviour
{
public PLCollection<string> characterResponses;
public string SelectResponse(string category)
{
var result = characterResponses.PickValueFrom(category, out var response);
if (result.ValueSelected)
{
return response;
}
if (result.ListFound)
{
Debug.Log($"No responses found for category {category}");
}
else
{
Debug.Log($"Category {category} not found in collection");
}
return string.Empty;
}
}
Next, create a new GameObject in the Unity Editor and attach the CharacterResponses
script to it.
You can start designing your collection of responses by adding Probability Lists to collection in the inspector.
Here, we added three lists of responses with the categories Friendly, Neutral, and Hostile.
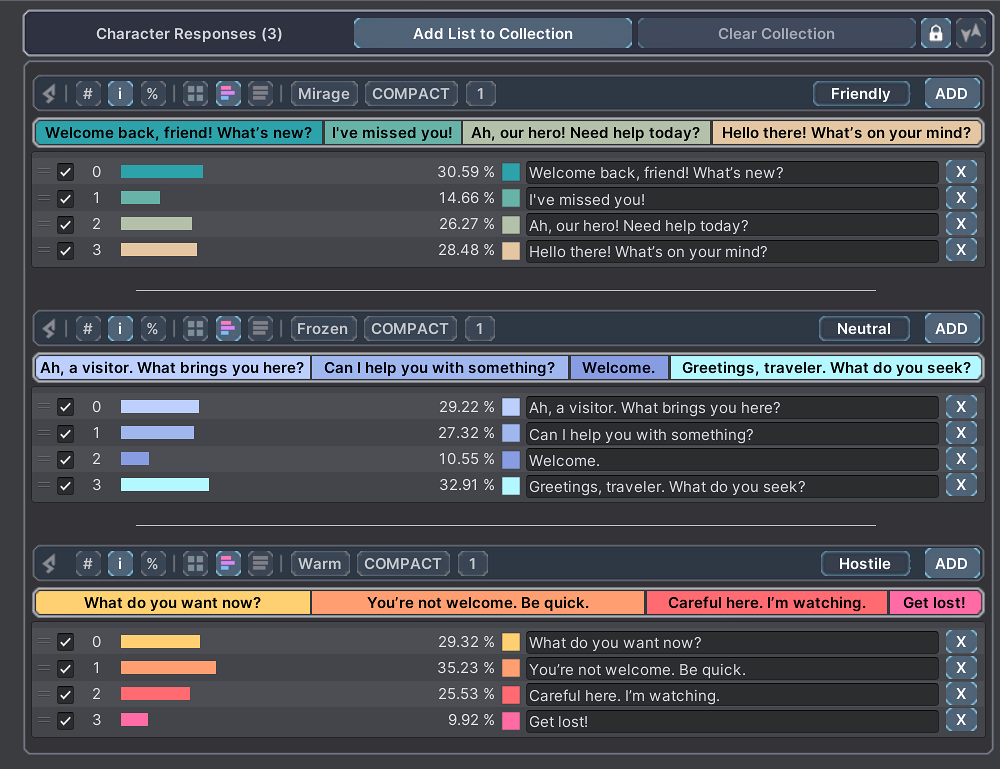
Now, you can call the SelectResponse
method to get a response from a specific category.
CharacterResponsesUsage.cs
Debug.Log(characterResponses.SelectResponse("Friendly")); // Output: "Welcome back, friend! What’s new?"
Debug.Log(characterResponses.SelectResponse("Neutral")); // Output: "WCan I help you with something?"
Debug.Log(characterResponses.SelectResponse("Hostile")); // Output: "Careful here. I’m watching."
For more complex use cases utilizing a modular loot table approach, check out the Treasure Chest and Deck Builder samples included with the plugin. Learn more about these and other examples on the Samples Overview.
Have a use case in mind that we should explore on this page? Join our Discord community and share your thoughts.